Animating Blur Radius
At last year's WWDC they announced an ability to animate blur radius during session 107 What's New in Cocoa Touch. It made developers very happy, but was never mentioned for the rest of the conference and kinda got forgotten.
Animated blur radius 👍🏽
— Ish (@ishabazz) June 9, 2015
During this year's session 228 A Peek at 3D Touch I noticed they had blur animations in the demo app so I quickly downloaded its code and had a peek at it. Turns out all they did was change UIVisualEffectViews's effect property to/from nil within an animation block. I thought “No way, could that be it? Is it possible that I missed this for an entire year?” And sure enough, I tried to build the same thing with Xcode 7 onto an iOS 9 device and it worked.
If you check out replies to above tweet from Ish, you will see this reply from me:
@ishabazz session 229 only mentions being able to animate the effect (between light/extraLight/dark styles) 😏
— Filip Radelic (@fichek) June 12, 2015
It was right there in front of me the whole time and I failed to see it. nil was actually a valid and animatable value for UIVisualEffectView's effect property. If only we had nullability as a hint to figure that out. :) But every year at WWDC we are showered with tons of new and improved APIs and it's not impossible to miss something cool even when you devote an entire week just to keeping up with all the news. That's why we need to talk to each other about cool stuff we learned that week, and it's one of the reasons I started this blog. Anyway…
iOS 10 and UIViewPropertyAnimator
This is where it gets really interesting. In iOS 10 you can easily make any UIKit animation user-driven using UIViewPropertyAnimator. That means you can now finally replicate that effect Springboard does when you pull down Spotlight's search bar and it gradually blurs as you get it closer to its final position. It's the very effect that was mentioned as an example of what we could do with blur radius animations when they were announced.
Here's an example of how to do an interactive blur. It's driven by a UISlider just to keep it short, but it's easy to drive the same thing with gesture recognizer, scroll view delegate or anything else you can imagine.
@IBOutlet weak var effectView: UIVisualEffectView!
var animator: UIViewPropertyAnimator?
override func viewDidLoad() {
super.viewDidLoad()
animator = UIViewPropertyAnimator(duration: 1, curve: .linear) {
self.effectView.effect = nil
}
}
@IBAction func sliderValueChanged(_ sender: UISlider) {
animator?.fractionComplete = CGFloat(sender.value)
}
Sample project is available on GitHub. To learn more about the awesome new UIViewPropertyAnimator, see session 216 Advances in UIKit Animations and Transitions. If you find something else this cool, please share it with fellow developers, there's a good chance some of us missed it :)
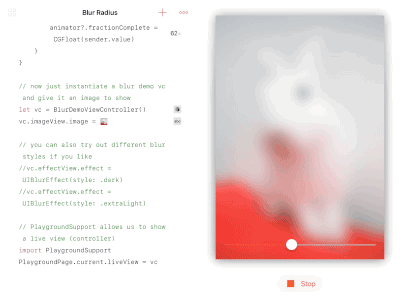
Update: I made a Swift Playground which is now included in Git repo. You can also download the playground directly.
It's a zip file because .playground is actually a folder which makes it a bit harder to get on your iPad directly from the Internet, but you can download it to your Mac, unzip and then either:
send it to your iPad via AirDropput it in iCloud Drive and import using + button in the app (you can do that even on a non-Mac computer via iCloud.com)send it via Mail (Mail app on iPad will tell you it can't open the attachment, but if you tap and hold, it will let you copy it to Playgrounds)
Please let me know on Twitter if you have a more elegant solution.
@fichek @ericasadun what if you download the zip on the iPad?
— Izzy (@frozendevil) June 21, 2016
@fichek @ericasadun IIRC Safari should "just know" what to do. If it doesn't I'd like to know :)
— Izzy (@frozendevil) June 21, 2016
Update 2: Turns out you can open the zip file directly on your iPad. It just needs to have .playground.zip extension and Safari will indeed "just know" what to do. Thanks Erica and Izzy.